ESP32 is a single 2.4 GHz Wi-Fi-and-Bluetooth combo chip designed with the TSMC low-power 40 nm technology.
We will use the GPIO pins functionality only in this post, but each pin has more than one possible functionality and while using a pin for particular task, we need to double check its alternative functions. Most of the GPIO pins have PWN functionality except pins (34,35,36,39), some GPIO pins (6-11) are used for SPI Flash IC . So, they shouldn’t be used for other purposes.
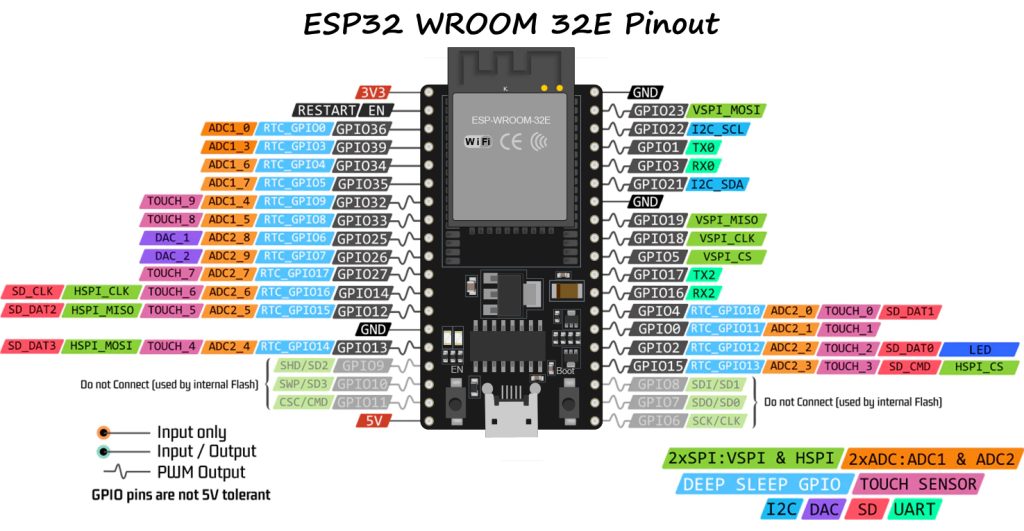
To install micropython on ESP32, I started with Thonny IDE https://www.thonny.org/. To install the micropython firmware on the ESP module, we have to download it from https://www.micropython.org/download/ and look for correct ESP32 module. Download the correct firmware version on your computer.
Next connect your module to your computer, and in Thonny under Tools -> Options select the micropython interpreter as ESP32, and from the Ports select your port where ESP32 is connected, and click on Install or update firmware. This will refresh the board and will install the firmware to support micro python.
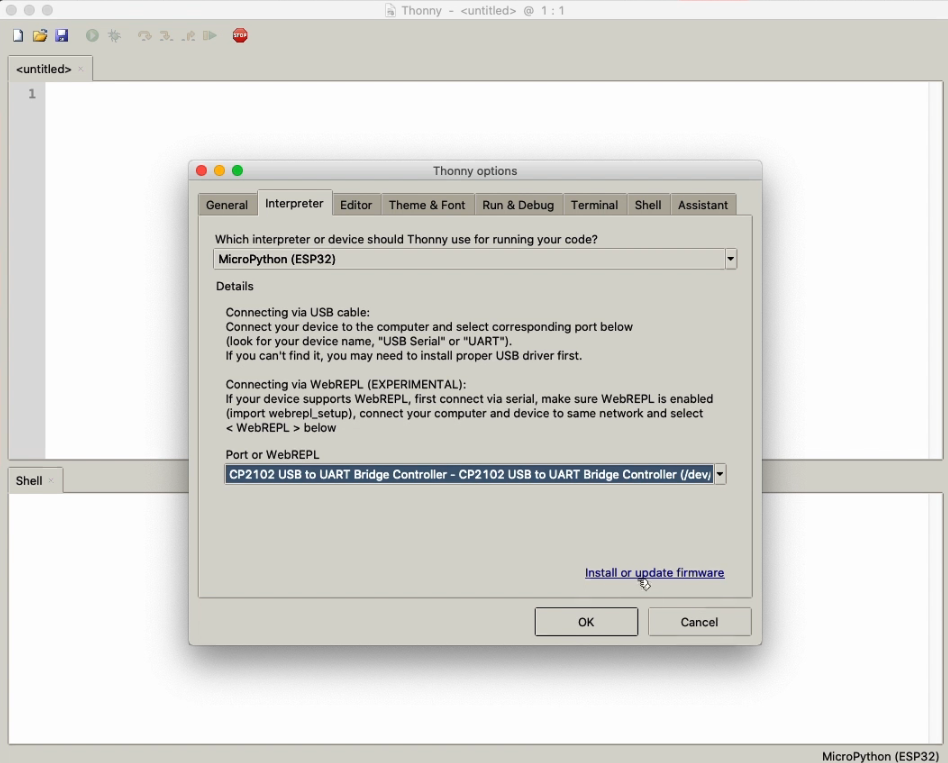
We will be building the following circuit.
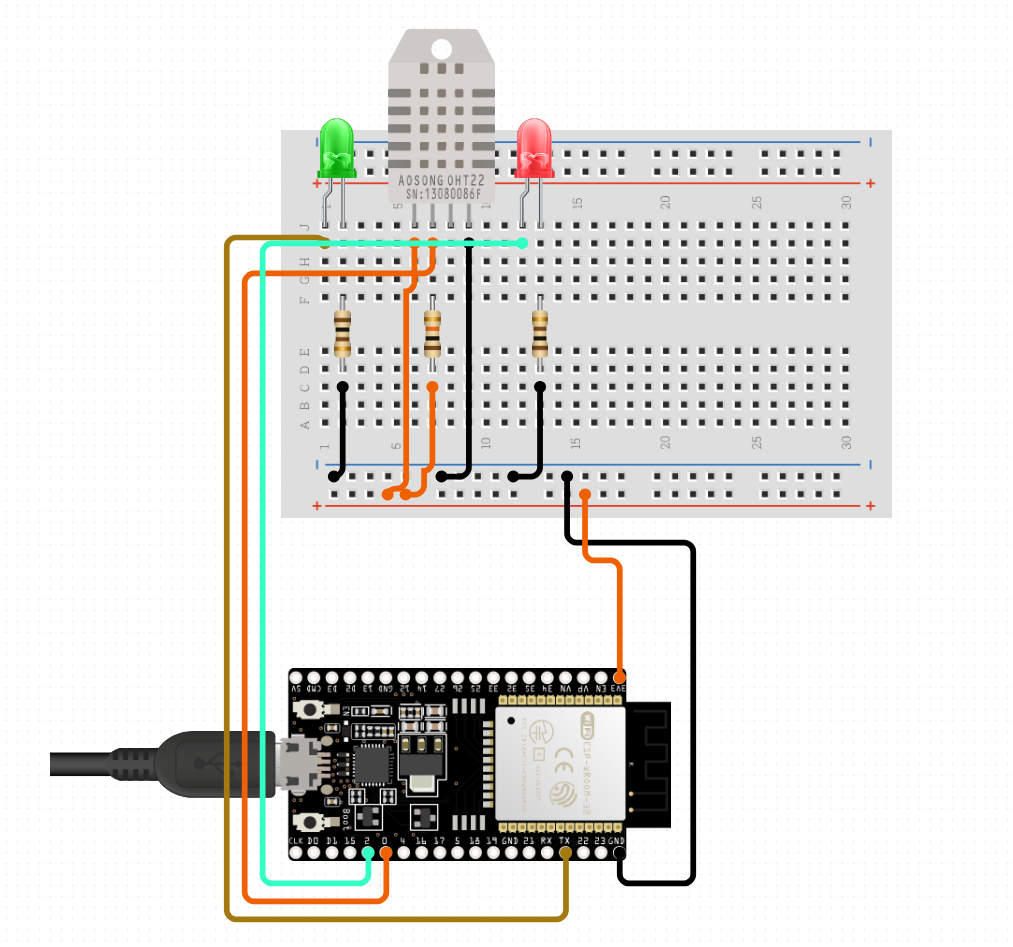
The DHT sensor is connected to PIN 0
The Green LED is connected to PIN 1
The Red LED is connected to PIN 2
We will read the temperature and humidity from the Pin 0 and will turn on the green LED if temperature is below 24°C and humidity is less than 50%, otherwise we will turn on the red LED
from machine import Pin, Timer
import dht
dht22 = dht.DHT22(Pin(0)) # dht pin on GPIO0
greenLed = Pin(1, Pin.OUT) # create output pin on GPIO1
redLed = Pin(2, Pin.OUT) # create output pin on GPIO2
'''
the measure statement triggers the read, and the values are fetched using the separate functions.
'''
def take_measurement_isr_and_alert(event):
dht22.measure()
temperature = dht22.temperature()
humidity = dht22.humidity()
print("Temp: ", temperature, "°C, Humidity: ", humidity, "%")
if temperature < 24 and humidity < 50:
greenLed.on()
redLed.off()
else:
greenLed.off()
redLed.on()
dht_timer = Timer(1)
dht_timer.init(period=5000, mode=Timer.PERIODIC, callback=take_measurement_isr_and_alert)
This code utilizes a non blocking Timer, we could have used a while loop with a sleep but that would block all other execution and we have to send a interrupt to do anything else.
Cheers – Amit Tomar !!!