We are going develop an iOS app today. We will run a small game of rock paper scissors which is easy to understand.
Let’s dive in.
Download Xcode and create a new app
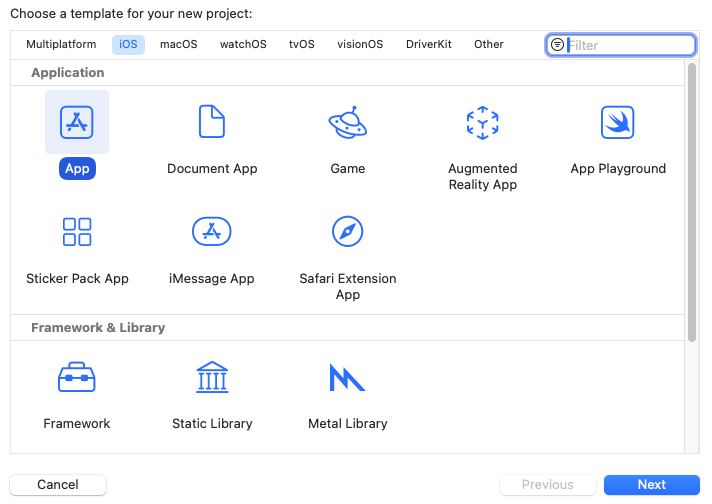
Give any name to you app and make sure you select Interface as “Storyboard”
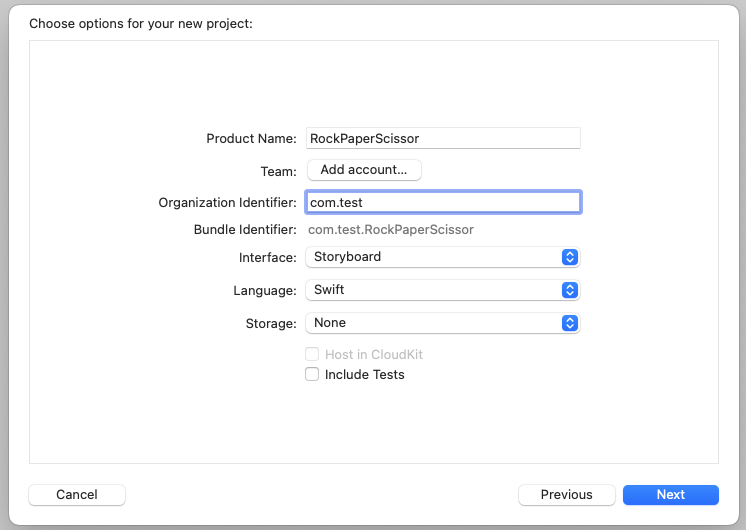
Put all the images in Assets folder
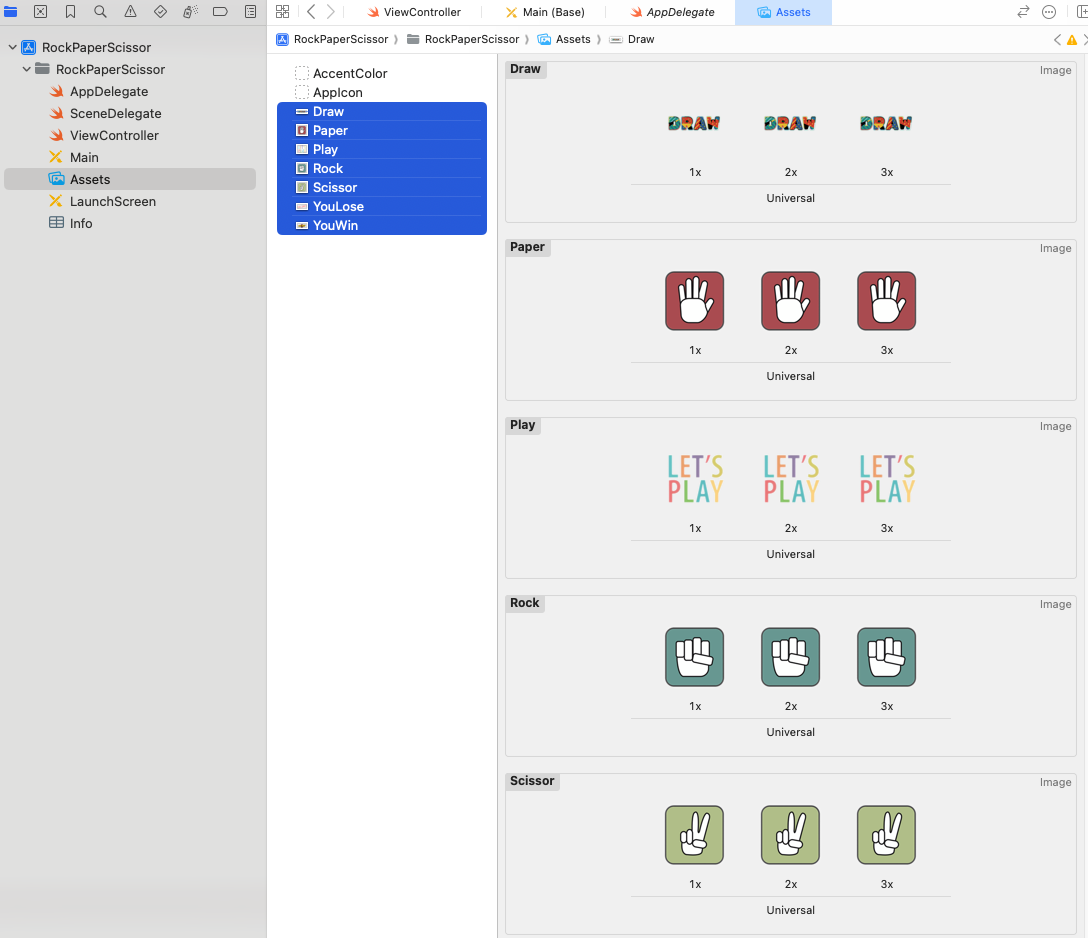
Arrange labels and images on the iPhone screen. (Select the Main story board from resources so you can see the iPhone simulator and your view controller side by side)
The top icons below CPU and Player are Image assets, but the bottom icons below “Make your move” are buttons with images on them
Notice that the view controller is still empty
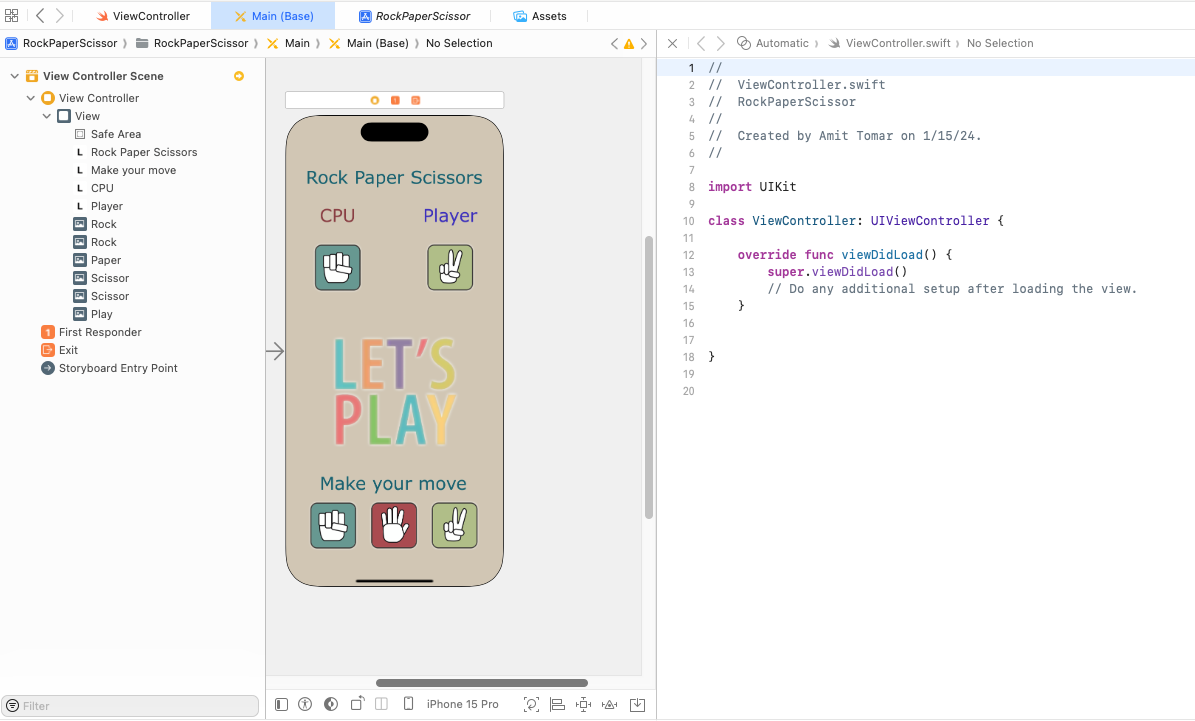
Do a Control+Click on the image icon on top and drag your cursor to view controller pane to the section where variables are defined
It will create a handle to the image object which can be used to update the image
Here I have already created cpuChoice variable and am creating playerChoice variable
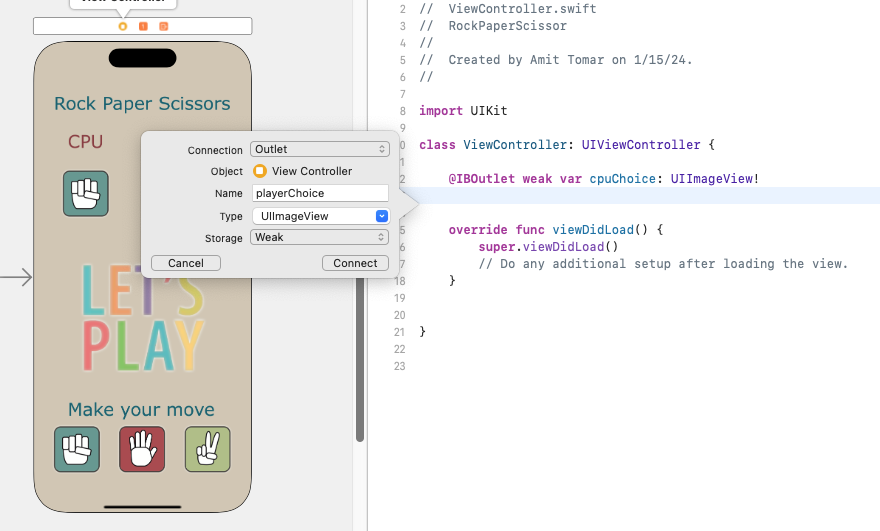
Do same thing with the button, but this time it will create an action “IBAction” which will be a function to be called when user taps on the button.
Here I have created the empty function for rock, and am creating an action for paper here.
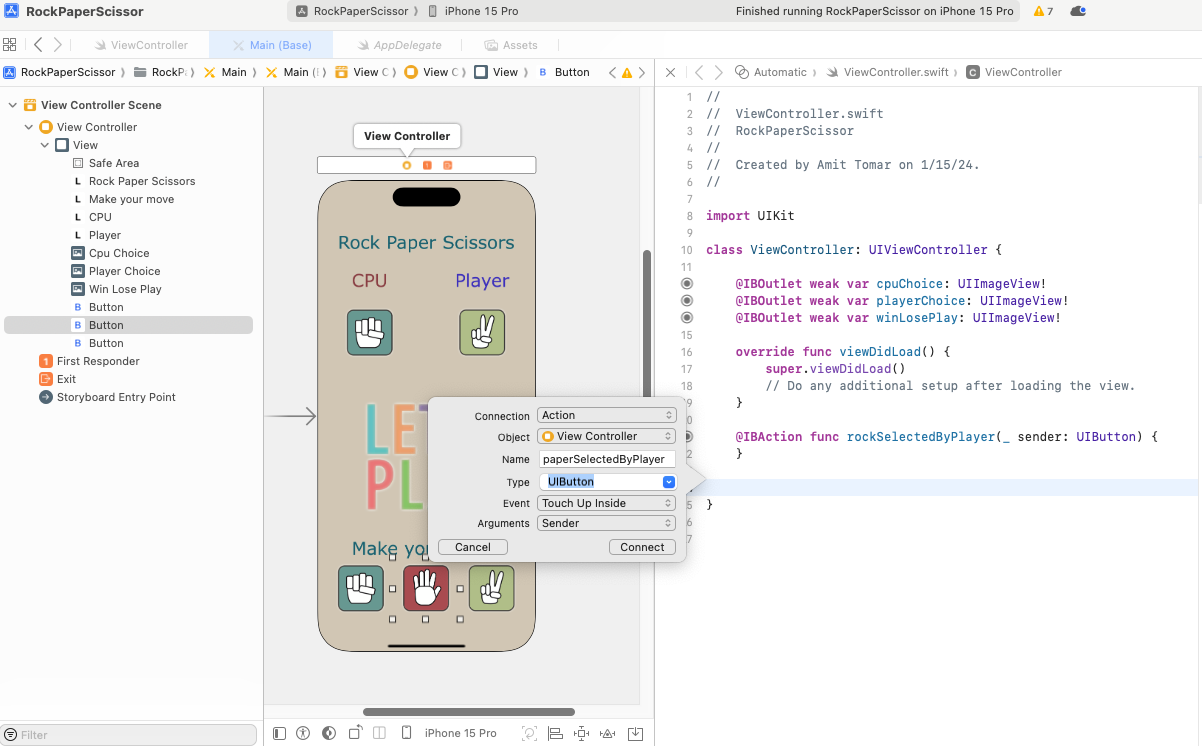
This is your code so far – All blank, but all connected to UI.
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var cpuChoice: UIImageView!
@IBOutlet weak var playerChoice: UIImageView!
@IBOutlet weak var winLosePlay: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func rockSelectedByPlayer(_ sender: UIButton) {
}
@IBAction func paperSelectedByPlayer(_ sender: UIButton) {
}
@IBAction func scissorsSelectedByPlayer(_ sender: UIButton) {
}
}
Now we have to write the business logic, when the button gets pressed,
- Identify which button was pressed.
- Let CPU make a choice
- Update the images on top
- Decide who won
- Update the middle image with result
- Wait for 1 second and reset screen for next game
//
// ViewController.swift
// RockPaperScissor
//
// Created by Amit Tomar on 1/15/24.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var cpuChoice: UIImageView!
@IBOutlet weak var playerChoice: UIImageView!
@IBOutlet weak var winLosePlay: UIImageView!
var choices = ["Rock", "Paper", "Scissor"]
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func rockSelectedByPlayer(_ sender: UIButton) {
playGame(playerChose: 0)
}
@IBAction func paperSelectedByPlayer(_ sender: UIButton) {
playGame(playerChose: 1)
}
@IBAction func scissorsSelectedByPlayer(_ sender: UIButton) {
playGame(playerChose: 2)
}
func playGame(playerChose: Int) {
let cpuChose = Int.random(in: 0...2)
playerChoice.image = UIImage(imageLiteralResourceName: choices[playerChose])
cpuChoice.image = UIImage(imageLiteralResourceName: choices[cpuChose])
if playerChose == 0 { //Rock
if cpuChose == 0 {//Rock
winLosePlay.image = UIImage(imageLiteralResourceName: "Draw")
} else if cpuChose == 1 { //Paper
winLosePlay.image = UIImage(imageLiteralResourceName: "YouLose")
} else { //Scissors
winLosePlay.image = UIImage(imageLiteralResourceName: "YouWin")
}
} else if playerChose == 1 { //Paper
if cpuChose == 0 {//Rock
winLosePlay.image = UIImage(imageLiteralResourceName: "YouWin")
} else if cpuChose == 1 { //Paper
winLosePlay.image = UIImage(imageLiteralResourceName: "Draw")
} else { //Scissors
winLosePlay.image = UIImage(imageLiteralResourceName: "YouLose")
}
} else { //Scissors
if cpuChose == 0 {//Rock
winLosePlay.image = UIImage(imageLiteralResourceName: "YouLose")
} else if cpuChose == 1 { //Paper
winLosePlay.image = UIImage(imageLiteralResourceName: "YouWin")
} else { //Scissors
winLosePlay.image = UIImage(imageLiteralResourceName: "Draw")
}
}
Timer.scheduledTimer(timeInterval: 1.0, target: self, selector: #selector(playAgain), userInfo: nil, repeats: false)
}
@objc func playAgain() {
winLosePlay.image = UIImage(imageLiteralResourceName: "Play")
}
}
And here are some screenshots of app in action
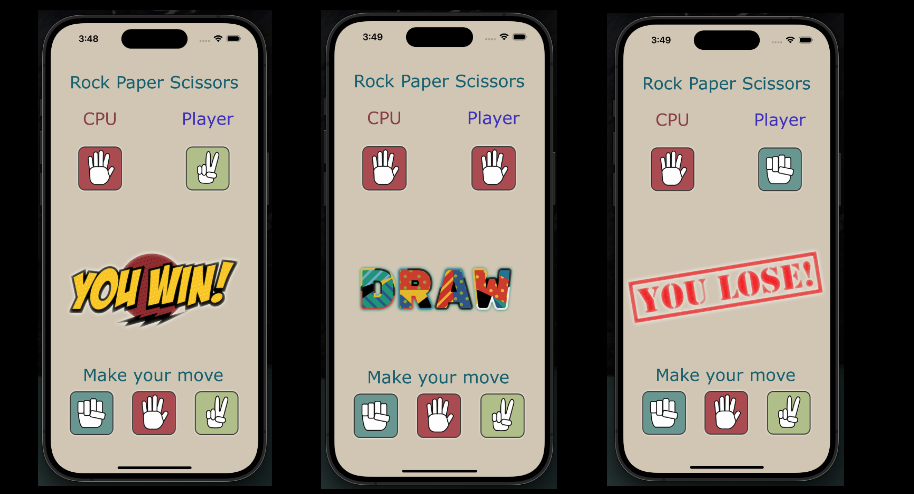
Pretty simple. Well there are some optimizations for responsive behavior (screen sizes, landscape mode etc) but they are also very straightforward
Cheers!! – Amit Tomar