Overview
Well you never know when the Doctor will show up in the neighborhood in his TARDIS. Its a hint that creatures from across the universe are going to cause trouble in England. Here a simple example will show how to identify if the Doctor is in town
Import for Google library
The face_recognition module commonly used in industry is developed by Adam Geitgey
import face_recognition
import numpy as np
from google.colab.patches import cv2_imshow
import cv2
Encode the known images of the Doctors and put the encodings in an array
# Encoding profiles for all doctors
face_1 = face_recognition.load_image_file("ChristopherEccleston.jpg")
face_1_encoding = face_recognition.face_encodings(face_1)[0]
face_2 = face_recognition.load_image_file("DavidTennant.jpg")
face_2_encoding = face_recognition.face_encodings(face_2)[0]
face_3 = face_recognition.load_image_file("MattSmith.jpg")
face_3_encoding = face_recognition.face_encodings(face_3)[0]
face_4 = face_recognition.load_image_file("PeterCapaldi.jpg")
face_4_encoding = face_recognition.face_encodings(face_4)[0]
face_5 = face_recognition.load_image_file("JodieWhittaker.jpg")
face_5_encoding = face_recognition.face_encodings(face_5)[0]
# all known face encodings and names
known_face_encodings = [face_1_encoding, face_2_encoding, face_3_encoding, face_4_encoding, face_5_encoding]
known_face_names = ["Christopher Eccleston", "David Tennant", "Matt Smith", "Peter Capaldi", "Jodie Whittaker"]
These are the images I used, their faces encoded in an array.
Christopher Eccleston – Ninth Doctor
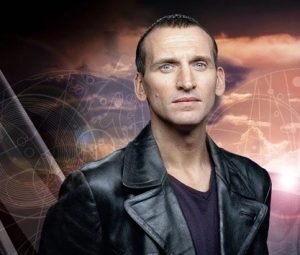
David Tennant – Tenth Doctor
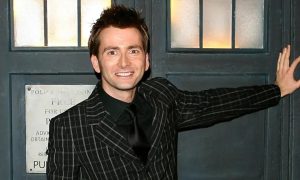
Matt Smith – Eleventh Doctor
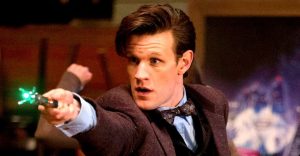
Peter Capaldi – Twelfth Doctor
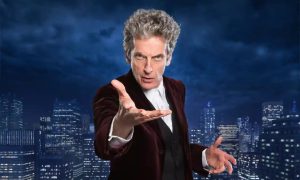
Jodie Whittaker – Thirteenth Doctor
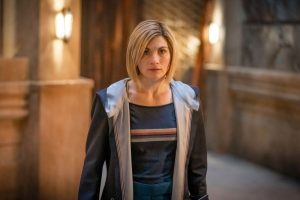
Load the image with some time lords in it
Well River Song was not a Doctor even though she was a part Time Lord. Here we identify the faces in the image and get their encodings.
# load the time lords image
file_name = "6TimeLords.jpg" t
ime_lords_image = face_recognition.load_image_file(file_name)
face_locations = face_recognition.face_locations(time_lords_image)
face_encodings = face_recognition.face_encodings(time_lords_image, face_locations)
# image on which we will write the names
time_lords_image_to_draw = cv2.imread(file_name)
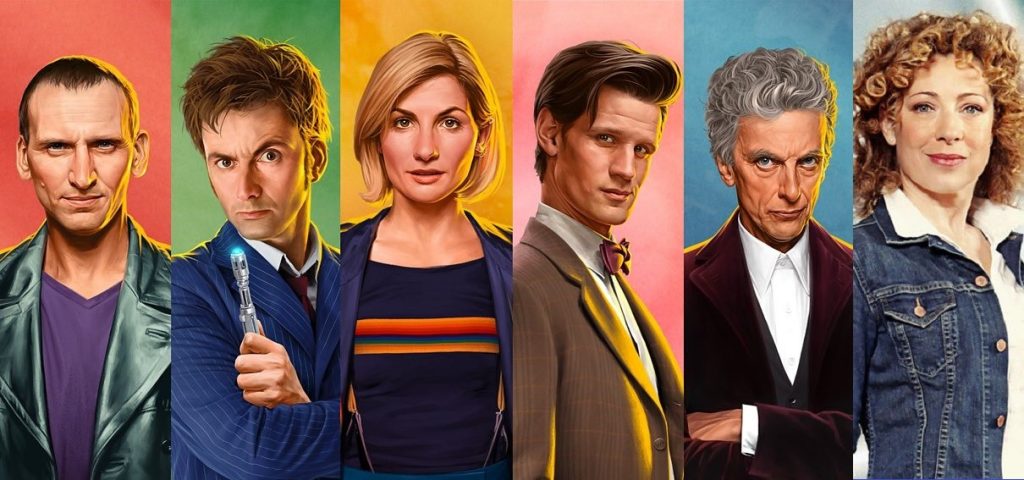
Now the matching algorithm
Identify the faces in the image, loop through encoded faces, and if we get a match then just draw a bounding box and name the doctor
#loop through faces identified
for (top,right, bottom, left), face_encoding in zip(face_locations, face_encodings):
name = "Not a Doctor"
# which face matched
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
face_distances = face_recognition.face_distance(known_face_encodings, face_encoding)
best_match_index = np.argmin(face_distances)
# if we get a good match, then get the name of the doctor
if matches[best_match_index]:
name = known_face_names[best_match_index]
# draw a rectangle across face and put the name
cv2.rectangle(time_lords_image_to_draw, (left, top), (right, bottom),(0,255,0),1)
cv2.putText(time_lords_image_to_draw,name, (left, bottom+20), cv2.FONT_HERSHEY_SIMPLEX,.5,(0,0,200),2, cv2.LINE_4)
#draw the final image
cv2_imshow(time_lords_image_to_draw)
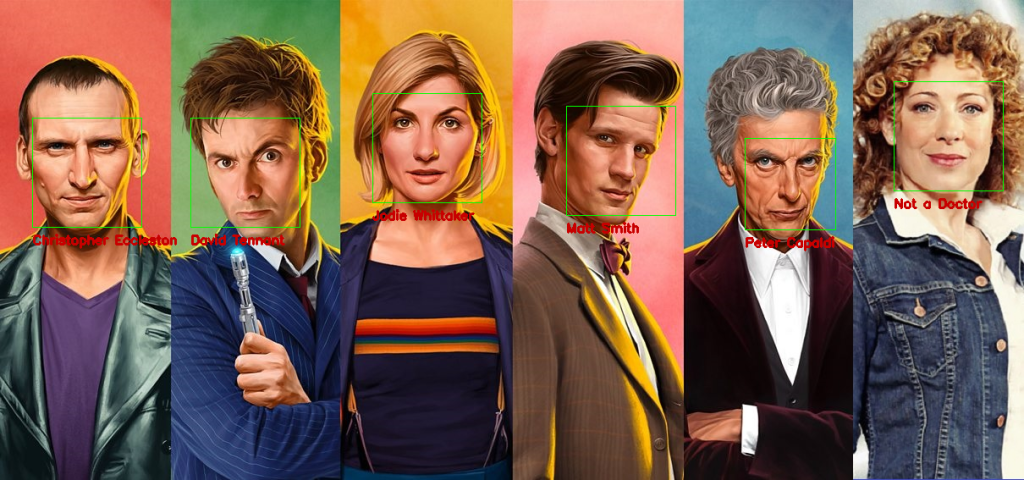
Here is the full code
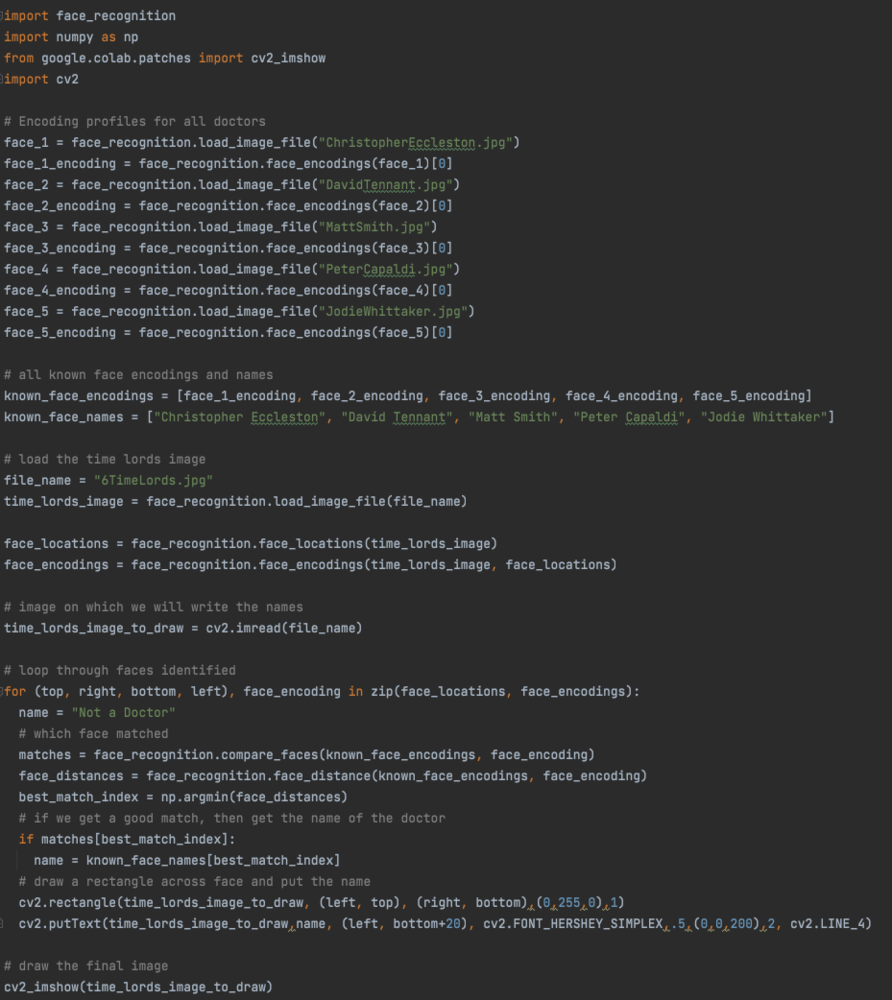
Cheers – Amit Tomar