Computer vision has great contour detection capabilities which when used with some other tools can yield beautiful results
Here is step by step process to get a image on which lane detection works.
Read the image
image = cv2.imread("images/road.jpg") cv2_imshow(image)
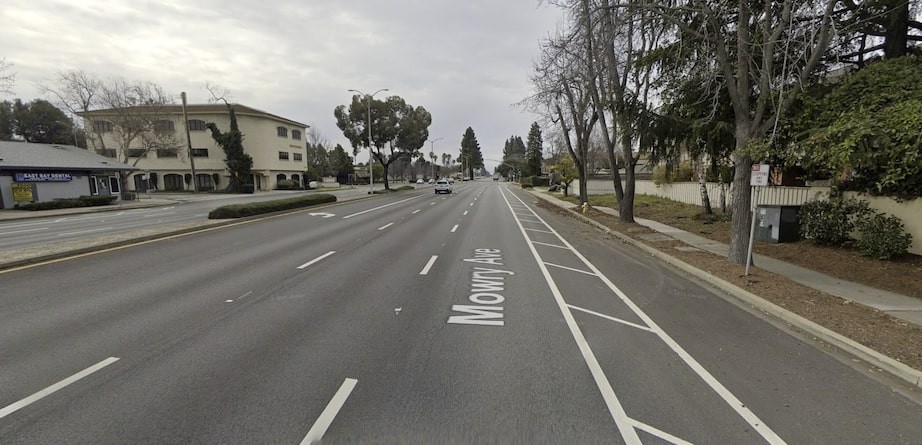
Convert the image to gray scale
gray = cv2.cvtColor(image,cv2.COLOR_BGR2GRAY) cv2_imshow(gray)
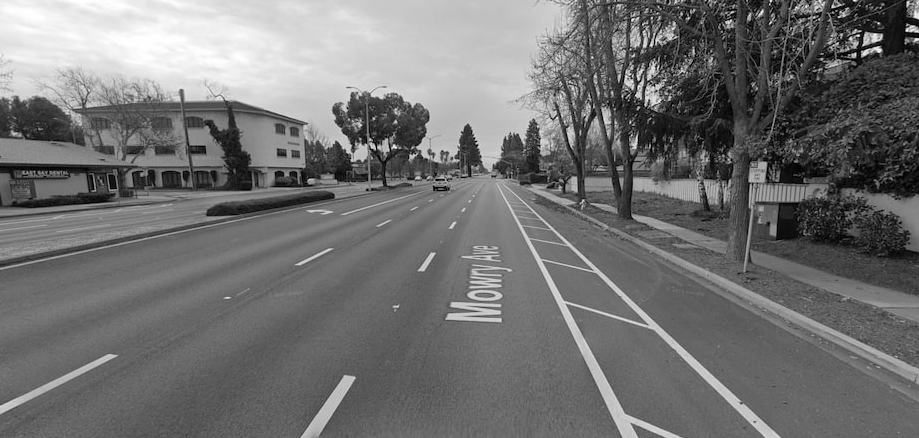
Create Canny image to show contours
canny_image = cv2.Canny(gray,150, 200) cv2_imshow(canny_image)
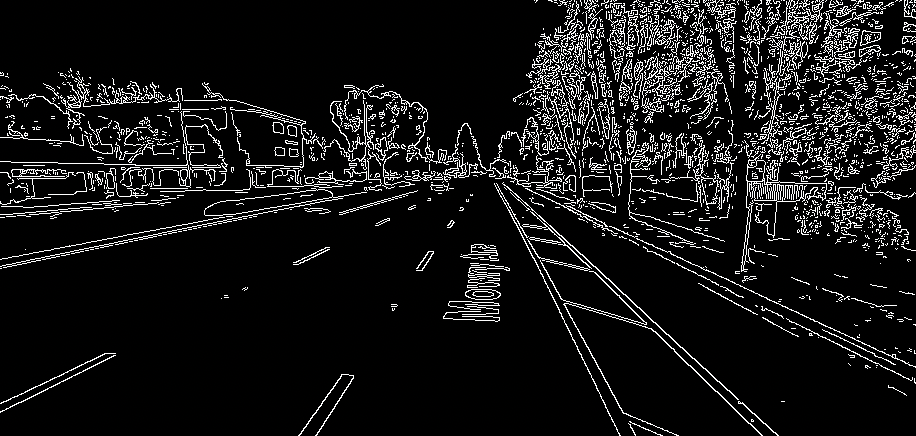
Dilate the contours to emphasize noise
kernel = np.ones((15, 15), np.uint8) # Dilation - emphasize the noise dilate_image = cv2.dilate(canny_image, kernel, iterations=1) cv2_imshow(dilate_image)
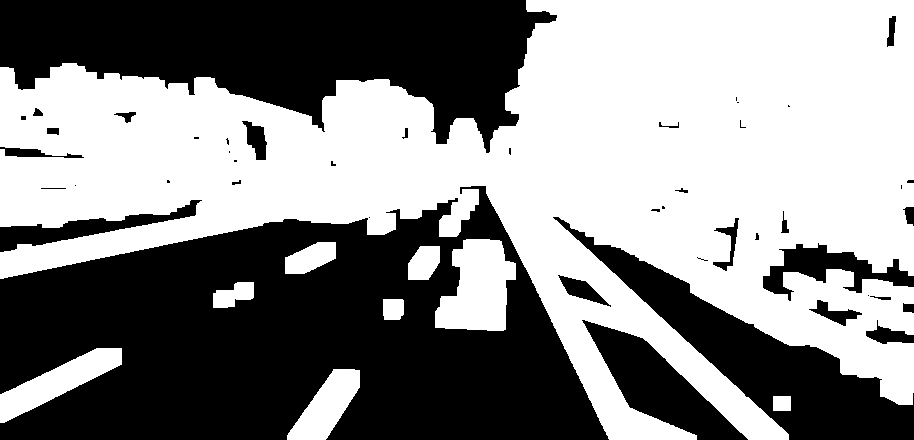
Erode the image to take out information
erode_image = cv2.erode(dilate_image,kernel, iterations=1) cv2_imshow(erode_image)
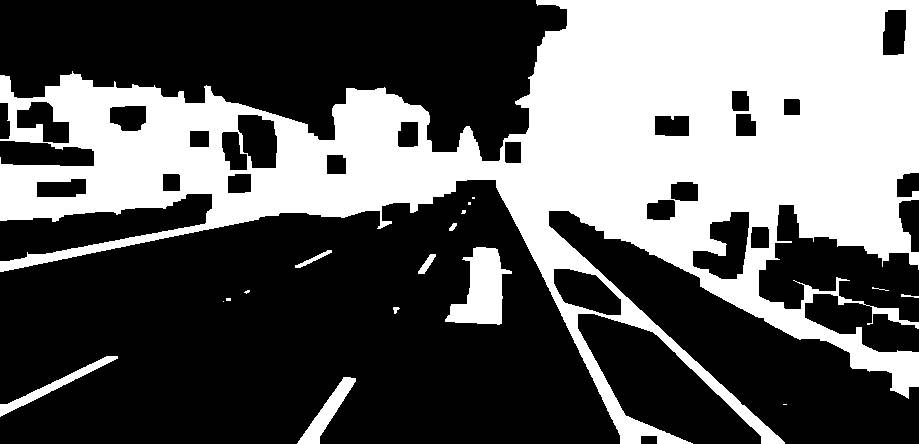
Create a canny image again to show final contours
canny_image = cv2.Canny(erode_image,150, 200) cv2_imshow(canny_image)
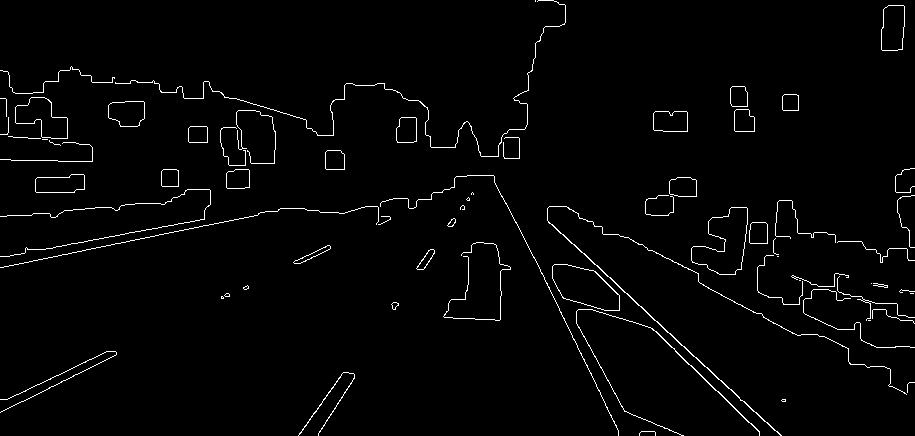
The whole code here
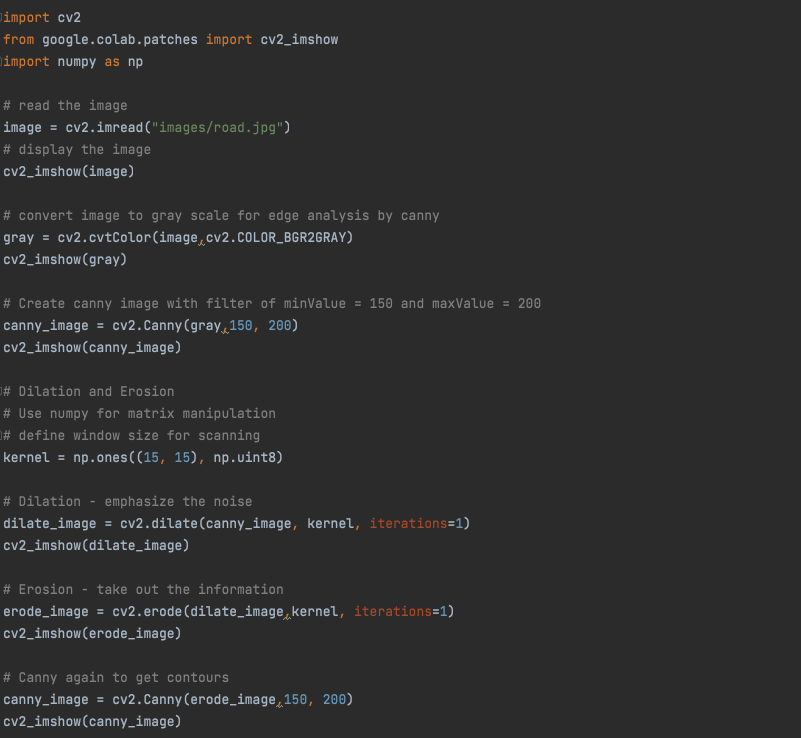
Cheers – Amit Tomar